The starting point for this project is the Kasa powerstrip I posted about, like a month ago. I’m trying o code up something to log power levels directly to a mysql db.
Both seem to have good documentation on how to use them.
The kasa library requires asyncio, which I haven’t really messed with async programing before, so I get to learn some new concepts. Though what little I know tells me it’s just a way to keep a connection open while waiting on the other end to respond.
asyncio is a library to write concurrent code using the async/await syntax.
asyncio is used as a foundation for multiple Python asynchronous frameworks that provide high-performance network and web-servers, database connection libraries, distributed task queues, etc.
https://docs.python.org/3/library/asyncio.html
This is really all I need to know, I think.
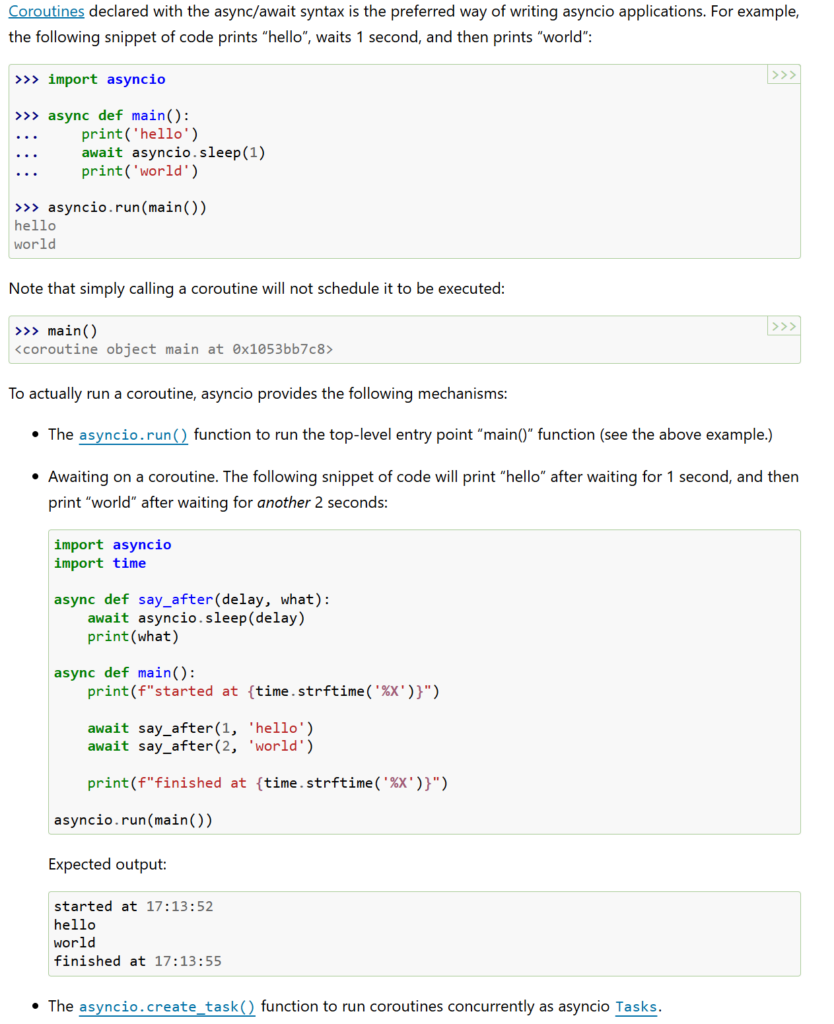
I’ve slapped together this to print the data I want with python
import asyncio
from kasa import Discover
async def main():
dev = await Discover.discover_single("powerstrip.lan")
await dev.update()
# possible features:
# state
# rssi
# on_since
# reboot
# led
# cloud_connection
# current_consumption
# consumption_today
# consumption_this_month
# consumption_total
# voltage
# current
state = dev.features.get("state")
voltage = dev.features.get("voltage")
current = dev.features.get("current")
current_consumption = dev.features.get("current_consumption")
consumption_this_month = dev.features.get("consumption_this_month")
consumption_today = dev.features.get("consumption_today")
# open file and write values
f = open("power.txt", "w")
f.write(f'Power On: {state.value}<br>{voltage.value} V {current.value} A {current_consumption.value} W<br>Today: {consumption_today.value} kwh<br>This Month: {consumption_this_month.value} kwh')
if __name__ == "__main__":
asyncio.run(main())
Which gives me this in WordPress:
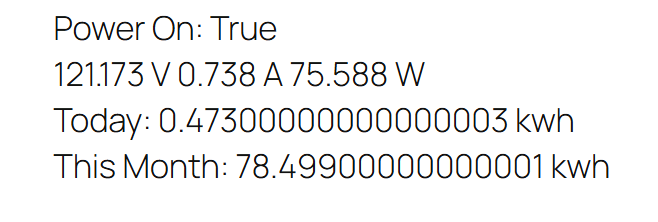
Not directly of course, I haven’t gotten that far yet. For now, the python writes a text file with HTML tags for display. I have an hourly cron job that uses scp to copy the file over to /var/www, and then some php in my functions.php file to load the file, and display it as a wordpress shortcode.
The stupid WordPress part took the longest, but I understand the layout of wordpress a little better. It has been around and popular for so long, that it is easy finding well written documentation, but I’ve found that a lot of it is outdated. It is very annoying to read through something, try it out, and then find your error is because you’re trying to use a deprecated feature.
The vision is a cron job that runs a python script to import data directly into the DB, and then php pulls the data from the DB, but the next step is to just get something into to DB.
Leave a Reply